Implementation of Eureka in spring boot

What is Eureka Server?
When we are working in a micro-service environment, Eureka plays an important role when it comes to service registry and discovery. Eureka holds a piece of information about registered services like their URL and port on which service is running. Another important feature of eureka is inter-service communication, we can directly communicate to another service using its eureka registry name. Thus, we don’t have to point actual address of the service and our application is independent of hard code service URLs.
Implementation of Eureka Server
Eureka has two entities:
· Eureka Server
· Eureka Client
Eureka Server
Step1: Create a New Project using Spring Initializer.
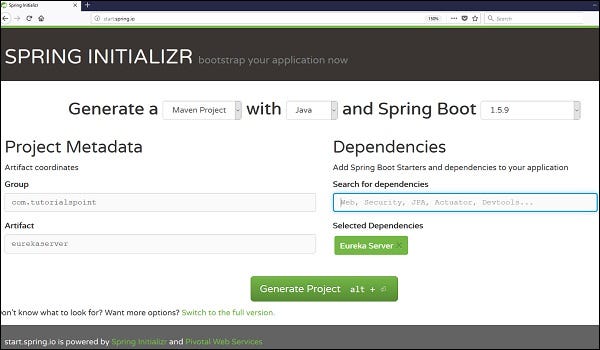
Step2: Add Required Dependency for Eureka Server:
For the maven project add this dependency in pom.xml:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
For the Gradle project add this dependency in the build.gradle:
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-server:${version}'
Step3: Annotate the Main class with the @EnableEurekaServer annotation
@EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {}
Step4: Add configuration to application.properties file:
server.port= 8083
eureka.client.register-with-eureka= false
eureka.client.fetch-registry = false
eureka.instance.prefer-ip-address=true
eureka.client.serviceUrl.defaultZone = http://localhost:8083/eureka
server.address=localhost
Step5: Run the application and open the URL http://localhost:8083/eureka in a browser. You will get outputs like:

Eureka Client
Step1: Create New Project using a spring initializer.
Step2: Add Required dependencies for Eureka Client.
For the maven project add this dependency in pom.xml:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
For the Gradle project add this dependency in the build.gradle:
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-:${version}'
Step3: Annotate the Main class with the @EnableEurekaClient annotation:
@EnableEurekaClient
@SpringBootApplication
public class ConsumerApplication {}
Step4: Add properties to application.properties:
spring.application.name = {service-name}
eureka.client.serviceUrl.defaultZone=http://{EUREKA_SERVER_URL}/eureka
eureka.client.instance.preferIpAddress = true
eureka.instance.hostname={host-name}
eureka.instance.prefer-ip-address=true
Step5: Run the Application and open eureka server Url on the browser:
